GolangでCognito認証しトークンを取得する
自分が関わっているサービスで、認証にAmazon Cognitoを使っています。
Amazon Cognito(ウェブ/モバイルアプリのユーザー管理)| AWS
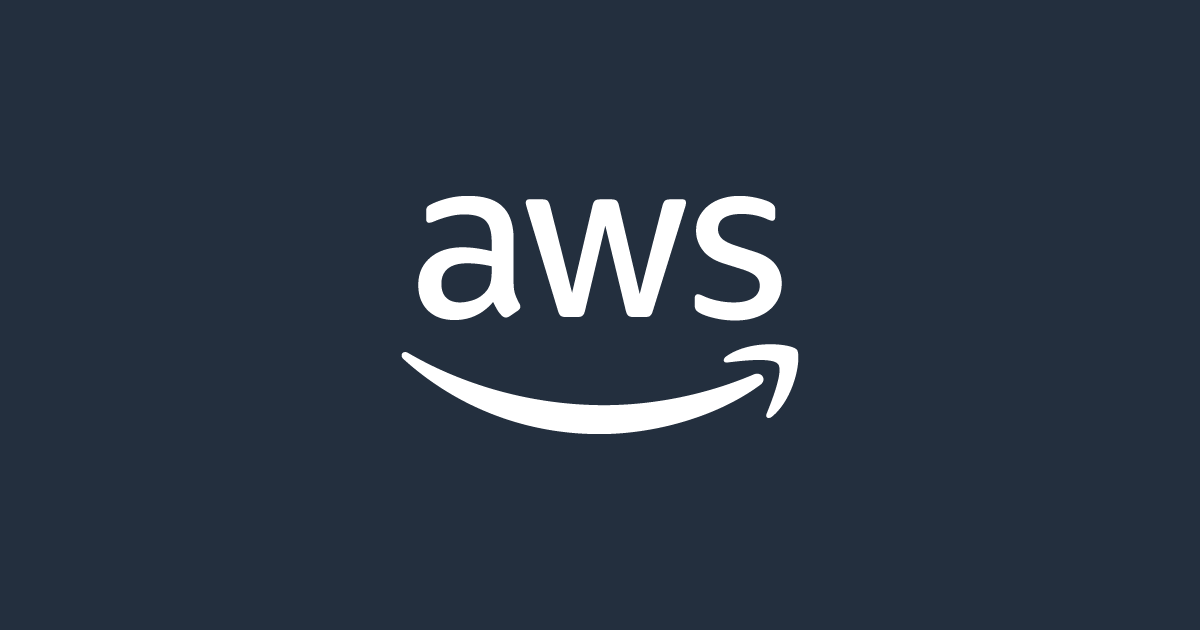
全てのリクエストについてトークンの検証をするので、APIサーバの動作確認時に必ずヘッダにトークンをセットする必要があります。このトークンを用意するのが地味に面倒だったので、Golangでスクリプトを書きました。
以下の記事を参考にしました。
golangでCognito認証(ただしSecure Remote Password(SRP)プロトコルではない) - Qiita
参考 http://docs.aws.amazon.com/ja_jp/cognito/latest/developerguide/amazon-cognito-user-pools-authentication-flow.ht...
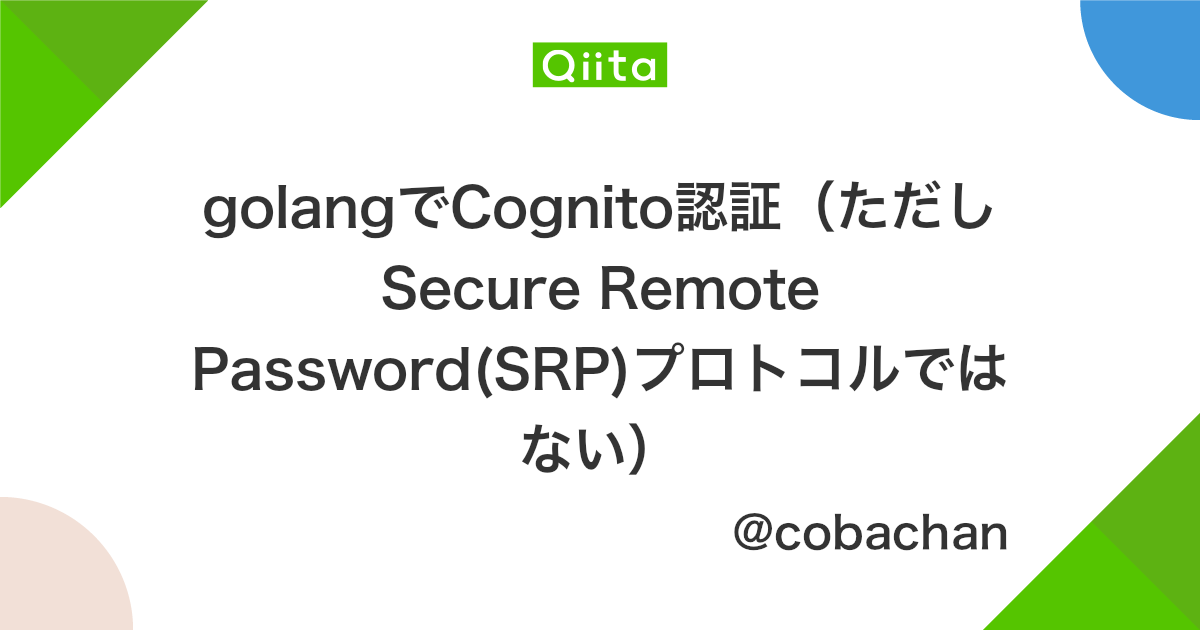
package main | |
import ( | |
"fmt" | |
"log" | |
"os" | |
"github.com/aws/aws-sdk-go/aws" | |
"github.com/aws/aws-sdk-go/aws/session" | |
"github.com/aws/aws-sdk-go/service/cognitoidentityprovider" | |
) | |
func main() { | |
if len(os.Args) != 3 { | |
log.Fatal("invalid args") | |
} | |
id := os.Args[1] | |
password := os.Args[2] | |
params := &cognitoidentityprovider.AdminInitiateAuthInput{ | |
AuthFlow: aws.String(cognitoidentityprovider.AuthFlowTypeAdminNoSrpAuth), | |
AuthParameters: map[string]*string{ | |
"USERNAME": aws.String(id), | |
"PASSWORD": aws.String(password), | |
}, | |
ClientId: aws.String(os.Getenv("CLIENT_ID")), | |
UserPoolId: aws.String(os.Getenv("USER_POOL_ID")), | |
} | |
client := cognitoidentityprovider.New( | |
session.Must(session.NewSessionWithOptions(session.Options{ | |
SharedConfigState: session.SharedConfigEnable, | |
})), | |
) | |
res, err := client.AdminInitiateAuth(params) | |
if err != nil { | |
log.Fatal(err) | |
} | |
if res == nil || res.AuthenticationResult == nil || res.AuthenticationResult.IdToken == nil { | |
log.Fatal("failed to login") | |
} | |
fmt.Println(*res.AuthenticationResult.IdToken) | |
} |
引数でusernameとpasswordを指定します。sessionの生成方法は自分の環境に合わせて少し変えています。以下を参考にしました。
Configuring the AWS SDK for Go - AWS SDK for Go
Configure the |sdk-go| to specify which credentials to use and to which AWS Region to send requests.
ローカルではDockerを使用しているので、簡単に使うためにMakefileに以下を追加します。
token:
docker-compose exec app go run main.go ${ID} ${PASS}
以下のように実行すると、トークンが出力されます。
$ make token ID=email@example.com PASS=password
以上です。